mirror of
https://github.com/mikefarah/yq.git
synced 2024-11-12 13:48:06 +00:00
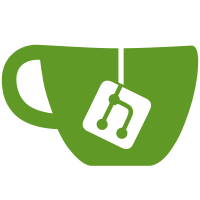
* Remove extra backtick * Reword explanation of update * Reword explanation of relative update * Change "remaple" to "remain" * Change "clovver" to "clobber" * Reword explanation of update for comment operators * Reword explanation of relative update for comment operators * Change "array" to "expression" * Change "the golangs" to "Golang's" * Change "golangs" to "Golang's" * Change "can durations" to "can add durations" * Change "array scalars" to "arrays" * Change "beit" to "be it" * Fix typo in `eval` tip * Fix typo in header for `has` operation * Add space before pipe in `line` operator example * Fix typos in explanation of deep array merges * Change "is now used" to "is now used." * Change "object," to "object." * Changes "indexes" to "indices" * Remove extraneous copied text from `..` article * Reword explanation of `...` operator * Change "your are" to "you are" * Add link to `string` operator docs in `select` article * Change "is a" to "parameter specifies" in `string` operators article * Change "new line" to "newline" * Change "golang regex" to "Golang's regex" * Change "golang" to "Golang" * Add period * Remove comma in `subtract` article * Remove duplicate number subtraction example * Remove comma in `traverse` operator article * Clarify use of brackets when `read`ing with special characters
271 lines
3.6 KiB
Markdown
271 lines
3.6 KiB
Markdown
# Assign (Update)
|
|
|
|
This operator is used to update node values. It can be used in either the:
|
|
|
|
### plain form: `=`
|
|
Which will set the LHS node values equal to the RHS node values. The RHS expression is run against the matching nodes in the pipeline.
|
|
|
|
### relative form: `|=`
|
|
This will do a similar thing to the plain form, but the RHS expression is run with _each LHS node as context_. This is useful for updating values based on old values, e.g. increment.
|
|
|
|
|
|
### Flags
|
|
- `c` clobber custom tags
|
|
|
|
## Create yaml file
|
|
Running
|
|
```bash
|
|
yq --null-input '.a.b = "cat" | .x = "frog"'
|
|
```
|
|
will output
|
|
```yaml
|
|
a:
|
|
b: cat
|
|
x: frog
|
|
```
|
|
|
|
## Update node to be the child value
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
a:
|
|
b:
|
|
g: foof
|
|
```
|
|
then
|
|
```bash
|
|
yq '.a |= .b' sample.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
a:
|
|
g: foof
|
|
```
|
|
|
|
## Double elements in an array
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
- 1
|
|
- 2
|
|
- 3
|
|
```
|
|
then
|
|
```bash
|
|
yq '.[] |= . * 2' sample.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
- 2
|
|
- 4
|
|
- 6
|
|
```
|
|
|
|
## Update node from another file
|
|
Note this will also work when the second file is a scalar (string/number)
|
|
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
a: apples
|
|
```
|
|
And another sample another.yml file of:
|
|
```yaml
|
|
b: bob
|
|
```
|
|
then
|
|
```bash
|
|
yq eval-all 'select(fileIndex==0).a = select(fileIndex==1) | select(fileIndex==0)' sample.yml another.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
a:
|
|
b: bob
|
|
```
|
|
|
|
## Update node to be the sibling value
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
a:
|
|
b: child
|
|
b: sibling
|
|
```
|
|
then
|
|
```bash
|
|
yq '.a = .b' sample.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
a: sibling
|
|
b: sibling
|
|
```
|
|
|
|
## Updated multiple paths
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
a: fieldA
|
|
b: fieldB
|
|
c: fieldC
|
|
```
|
|
then
|
|
```bash
|
|
yq '(.a, .c) = "potato"' sample.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
a: potato
|
|
b: fieldB
|
|
c: potato
|
|
```
|
|
|
|
## Update string value
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
a:
|
|
b: apple
|
|
```
|
|
then
|
|
```bash
|
|
yq '.a.b = "frog"' sample.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
a:
|
|
b: frog
|
|
```
|
|
|
|
## Update string value via |=
|
|
Note there is no difference between `=` and `|=` when the RHS is a scalar
|
|
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
a:
|
|
b: apple
|
|
```
|
|
then
|
|
```bash
|
|
yq '.a.b |= "frog"' sample.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
a:
|
|
b: frog
|
|
```
|
|
|
|
## Update deeply selected results
|
|
Note that the LHS is wrapped in brackets! This is to ensure we don't first filter out the yaml and then update the snippet.
|
|
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
a:
|
|
b: apple
|
|
c: cactus
|
|
```
|
|
then
|
|
```bash
|
|
yq '(.a[] | select(. == "apple")) = "frog"' sample.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
a:
|
|
b: frog
|
|
c: cactus
|
|
```
|
|
|
|
## Update array values
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
- candy
|
|
- apple
|
|
- sandy
|
|
```
|
|
then
|
|
```bash
|
|
yq '(.[] | select(. == "*andy")) = "bogs"' sample.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
- bogs
|
|
- apple
|
|
- bogs
|
|
```
|
|
|
|
## Update empty object
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
{}
|
|
```
|
|
then
|
|
```bash
|
|
yq '.a.b |= "bogs"' sample.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
a:
|
|
b: bogs
|
|
```
|
|
|
|
## Update node value that has an anchor
|
|
Anchor will remain
|
|
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
a: &cool cat
|
|
```
|
|
then
|
|
```bash
|
|
yq '.a = "dog"' sample.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
a: &cool dog
|
|
```
|
|
|
|
## Update empty object and array
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
{}
|
|
```
|
|
then
|
|
```bash
|
|
yq '.a.b.[0] |= "bogs"' sample.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
a:
|
|
b:
|
|
- bogs
|
|
```
|
|
|
|
## Custom types are maintained by default
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
a: !cat meow
|
|
b: !dog woof
|
|
```
|
|
then
|
|
```bash
|
|
yq '.a = .b' sample.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
a: !cat woof
|
|
b: !dog woof
|
|
```
|
|
|
|
## Custom types: clobber
|
|
Use the `c` option to clobber custom tags
|
|
|
|
Given a sample.yml file of:
|
|
```yaml
|
|
a: !cat meow
|
|
b: !dog woof
|
|
```
|
|
then
|
|
```bash
|
|
yq '.a =c .b' sample.yml
|
|
```
|
|
will output
|
|
```yaml
|
|
a: !dog woof
|
|
b: !dog woof
|
|
```
|
|
|